#include <koagendaview.h>
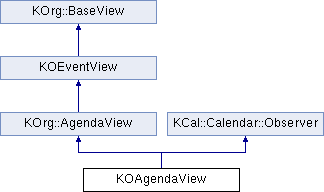
Public Slots | |
virtual void | updateView () |
virtual void | updateConfig () |
virtual void | showDates (const TQDate &start, const TQDate &end) |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date) |
void | insertIncidence (Incidence *incidence, const TQDate &curDate) |
void | changeIncidenceDisplayAdded (Incidence *incidence) |
void | changeIncidenceDisplay (Incidence *incidence, int mode) |
void | clearSelection () |
void | startDrag (Incidence *) |
void | readSettings () |
void | readSettings (TDEConfig *) |
void | writeSettings (TDEConfig *) |
void | setContentsPos (int y) |
void | setExpandedButton (bool expanded) |
void | finishTypeAhead () |
void | slotTodoDropped (Todo *, const TQPoint &, bool) |
void | enableAgendaUpdate (bool enable) |
void | setIncidenceChanger (KOrg::IncidenceChangerBase *changer) |
void | zoomInHorizontally (const TQDate &date=TQDate()) |
void | zoomOutHorizontally (const TQDate &date=TQDate()) |
void | zoomInVertically () |
void | zoomOutVertically () |
void | zoomView (const int delta, const TQPoint &pos, const TQt::Orientation orient=TQt::Horizontal) |
void | clearTimeSpanSelection () |
void | resourcesChanged () |
![]() | |
virtual void | finishTypeAhead ()=0 |
virtual void | resourcesChanged ()=0 |
![]() | |
void | defaultAction (Incidence *) |
![]() | |
virtual void | showDates (const TQDate &start, const TQDate &end)=0 |
virtual void | showIncidences (const Incidence::List &incidenceList, const TQDate &date)=0 |
virtual void | updateView ()=0 |
virtual void | dayPassed (const TQDate &) |
virtual void | setIncidenceChanger (IncidenceChangerBase *changer) |
virtual void | flushView () |
virtual void | changeIncidenceDisplay (Incidence *, int)=0 |
virtual void | updateConfig () |
virtual void | clearSelection () |
Signals | |
void | toggleExpand () |
void | zoomViewHorizontally (const TQDate &, int count) |
void | timeSpanSelectionChanged () |
![]() | |
void | datesSelected (const DateList) |
void | shiftedEvent (const TQDate &olddate, const TQDate &newdate) |
![]() | |
void | incidenceSelected (Incidence *, const TQDate &) |
void | showIncidenceSignal (Incidence *, const TQDate &) |
void | editIncidenceSignal (Incidence *, const TQDate &) |
void | deleteIncidenceSignal (Incidence *) |
void | cutIncidenceSignal (Incidence *) |
void | copyIncidenceSignal (Incidence *) |
void | pasteIncidenceSignal () |
void | toggleAlarmSignal (Incidence *) |
void | dissociateOccurrenceSignal (Incidence *, const TQDate &) |
void | dissociateFutureOccurrenceSignal (Incidence *, const TQDate &) |
void | startMultiModify (const TQString &) |
void | endMultiModify () |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &) |
void | newEventSignal (ResourceCalendar *res, const TQString &subResource, const TQDateTime &, const TQDateTime &) |
void | newTodoSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
void | newSubTodoSignal (Todo *) |
void | newJournalSignal (ResourceCalendar *res, const TQString &subResource, const TQDate &) |
Public Member Functions | |
KOAgendaView (Calendar *cal, CalendarView *calendarView, TQWidget *parent=0, const char *name=0, bool isSideBySide=false) | |
virtual int | maxDatesHint () |
virtual int | currentDateCount () |
virtual Incidence::List | selectedIncidences () |
virtual DateList | selectedIncidenceDates () |
virtual bool | eventDurationHint (TQDateTime &startDt, TQDateTime &endDt, bool &allDay) |
void | clearView () |
KOrg::CalPrinterBase::PrintType | printType () |
TQDateTime | selectionStart () |
TQDateTime | selectionEnd () |
bool | selectedIsAllDay () |
void | deleteSelectedDateTime () |
bool | selectedIsSingleCell () |
void | setTypeAheadReceiver (TQObject *) |
KOAgenda * | agenda () const |
TQSplitter * | splitter () const |
TQFrame * | dayLabels () const |
void | calendarIncidenceAdded (Incidence *incidence) |
void | calendarIncidenceChanged (Incidence *incidence) |
void | calendarIncidenceDeleted (Incidence *incidence) |
![]() | |
AgendaView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
![]() | |
KOEventView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~KOEventView () |
KOEventPopupMenu * | eventPopup () |
TQPopupMenu * | newEventPopup () |
bool | isEventView () |
bool | supportsDateNavigation () const |
![]() | |
BaseView (Calendar *cal, TQWidget *parent=0, const char *name=0) | |
virtual | ~BaseView () |
void | setReadOnly (bool readonly) |
bool | readOnly () |
virtual void | setCalendar (Calendar *cal) |
virtual Calendar * | calendar () |
virtual void | setResource (ResourceCalendar *res, const TQString &subResource) |
ResourceCalendar * | resourceCalendar () |
TQString | subResourceCalendar () const |
![]() | |
virtual void | calendarModified (bool, Calendar *) |
Protected Slots | |
void | updateEventDates (KOAgendaItem *item) |
void | doUpdateItem () |
void | updateEventIndicatorTop (int newY) |
void | updateEventIndicatorBottom (int newY) |
void | newTimeSpanSelected (const TQPoint &start, const TQPoint &end) |
void | newTimeSpanSelectedAllDay (const TQPoint &start, const TQPoint &end) |
void | updateDayLabelSizes () |
![]() | |
void | popupShow () |
void | popupEdit () |
void | popupDelete () |
void | popupCut () |
void | popupCopy () |
virtual void | showNewEventPopup () |
Protected Member Functions | |
void | fillAgenda (const TQDate &startDate) |
void | fillAgenda () |
void | connectAgenda (KOAgenda *agenda, TQPopupMenu *popup, KOAgenda *otherAgenda) |
void | createDayLabels (bool force) |
void | setHolidayMasks () |
void | removeIncidence (Incidence *) |
void | updateEventIndicators () |
void | updateTimeBarWidth () |
virtual void | resizeEvent (TQResizeEvent *resizeEvent) |
Additional Inherited Members | |
![]() | |
Incidence * | mCurrentIncidence |
![]() | |
IncidenceChangerBase * | mChanger |
Detailed Description
KOAgendaView is the agenda-like view used to display events in a single one or multi-day view.
Definition at line 108 of file koagendaview.h.
Member Function Documentation
◆ clearView()
void KOAgendaView::clearView | ( | ) |
Remove all events from view.
Definition at line 1517 of file koagendaview.cpp.
◆ createDayLabels()
|
protected |
Create labels for the selected dates.
Definition at line 599 of file koagendaview.cpp.
◆ currentDateCount()
|
virtual |
Returns number of currently shown dates.
Implements KOrg::BaseView.
Definition at line 699 of file koagendaview.cpp.
◆ deleteSelectedDateTime()
void KOAgendaView::deleteSelectedDateTime | ( | ) |
make selected start/end invalid
Definition at line 1697 of file koagendaview.cpp.
◆ doUpdateItem
|
protectedslot |
update just the display of the given incidence, called by a single-shot timer
Definition at line 1142 of file koagendaview.cpp.
◆ eventDurationHint()
|
virtual |
return the default start/end date/time for new events
Reimplemented from KOrg::BaseView.
Definition at line 732 of file koagendaview.cpp.
◆ fillAgenda() [1/2]
|
protected |
Fill agenda using the current set value for the start date.
Definition at line 1359 of file koagendaview.cpp.
◆ fillAgenda() [2/2]
|
protected |
Fill agenda beginning with date startDate.
Definition at line 1354 of file koagendaview.cpp.
◆ maxDatesHint()
|
virtual |
Returns maximum number of days supported by the koagendaview.
Implements KOEventView.
Definition at line 693 of file koagendaview.cpp.
◆ newTimeSpanSelected
|
protectedslot |
Updates data for selected timespan.
Definition at line 1678 of file koagendaview.cpp.
◆ newTimeSpanSelectedAllDay
|
protectedslot |
Updates data for selected timespan for all day event.
Definition at line 1672 of file koagendaview.cpp.
◆ selectedIncidenceDates()
|
virtual |
returns the currently selected events
Implements KOrg::BaseView.
Definition at line 718 of file koagendaview.cpp.
◆ selectedIncidences()
|
virtual |
returns the currently selected events
Implements KOrg::BaseView.
Definition at line 704 of file koagendaview.cpp.
◆ selectedIsAllDay()
|
inline |
returns true if selection is for whole day
Definition at line 145 of file koagendaview.h.
◆ selectedIsSingleCell()
bool KOAgendaView::selectedIsSingleCell | ( | ) |
returns if only a single cell is selected, or a range of cells
Definition at line 757 of file koagendaview.cpp.
◆ selectionEnd()
|
inlinevirtual |
end-datetime of selection
Reimplemented from KOrg::BaseView.
Definition at line 143 of file koagendaview.h.
◆ selectionStart()
|
inlinevirtual |
start-datetime of selection
Reimplemented from KOrg::BaseView.
Definition at line 141 of file koagendaview.h.
◆ setHolidayMasks()
|
protected |
Set the masks on the agenda widgets indicating, which days are holidays.
Definition at line 1629 of file koagendaview.cpp.
◆ slotTodoDropped
|
slot |
reschedule the todo to the given x- and y- coordinates.
Third parameter determines all-day (no time specified)
Definition at line 1548 of file koagendaview.cpp.
◆ updateEventDates
|
protectedslot |
Update event belonging to agenda item.
Definition at line 846 of file koagendaview.cpp.
◆ updateEventIndicators()
|
protected |
Updates the event indicators after a certain incidence was modified or removed.
Definition at line 1722 of file koagendaview.cpp.
The documentation for this class was generated from the following files: